This post is my contribution to Azure Back to School 2020, a fantastic idea that was created by Dwayne Natwick. It gives a chance for all the #AzureFamily members to contribute a video or write a blog post about any Azure topic for every day of September.
Be sure to follow along with the upcomming articles from #AzureBackToSchool!
In this part of a multi-series blog about Infrastructure as Code, I’m going to explain why a new project like Bicep is currently in need to build your Azure Infrastructure.
Why Bicep?
If you’ve ever worked with ARM Templates, you know it’s hard to learn the JSON language, (the current ARM Template syntax) and write complex templates.
Let’s face it, the problem with ARM Templates and the ARM syntax is the learning curve. Learning to write ARM Templates is painful.
Like any other new skill you learn, in the beginning, it’s going to hurt. That’s the same with writing templates. The first 20 ARM Templates are painful, after you’ve created 100 or more, it is going to be OK and more fun.
Keep in mind that there will always be some complexity about ARM Templates. For example, they are not that nice to scale. If you want to share templates between different teams, it is difficult because every team member has his own method of writing templates.
So first there was a joke about ARM, now there is a bicep?
What is Bicep?
It’s a Domain Specific Language (DSL) for deploying Azure resources declaratively. DSLs are small languages, focused on a particular aspect of a software system. It is meant for use in the context of a particular domain. A domain could be a business context (e.g., banking, insurance, etc.) or an application context (web application, database, etc.). A good example of a DSL is HTML.
Bicep is a new thing and is not production ready today. So play with it and submit feedback, because it is going to change as it is developed along the way.
Bicep is just released and it will be evolving fast.
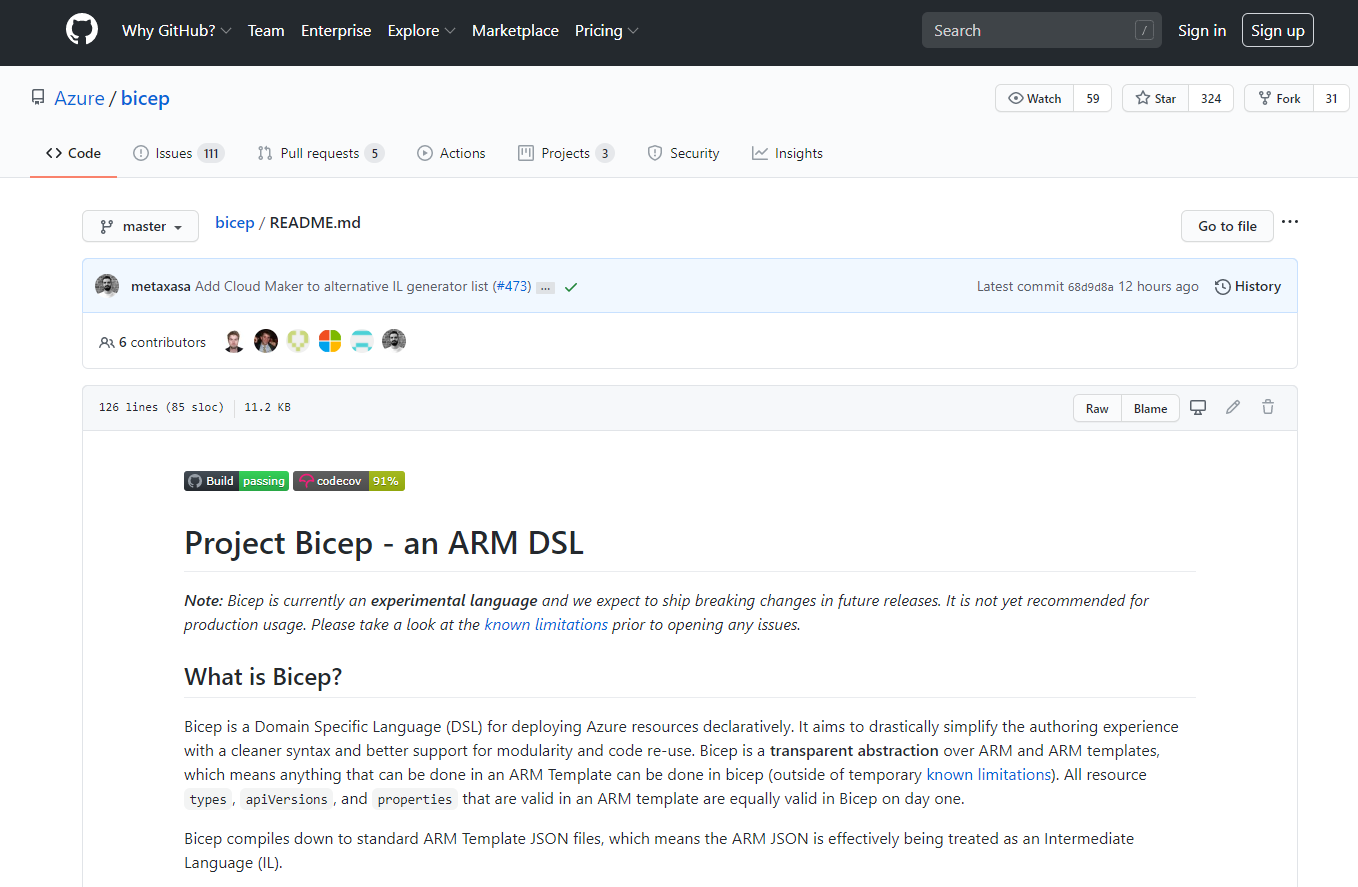
The first thing you have to know, is that Bicep is a transparent abstraction layer over ARM and it is not a re-write of ARM templates.
Anything you can do with ARM you can do with bicep and anything you can do with bicep, you can do with ARM.
The only difference is how easy you can do certain things with Bicep. It aims to drastically simplify the authoring experience with a cleaner syntax.
You write the bicep files and compile those into ARM Template JSON files. So you take bicep files in and ARM templates out. Simple and easy!
In the same way as you do with ARM generation (you can always export a ARM template from the portal) you can have bad quality templates. The same thing is true for bicep files. If you generate bicep files from ARM Templates, they are not nice.
Bicep in short
- Domain Specific Language (DSL) for deploying Azure resources declaratively
- Bicep is a transparent abstraction over ARM and ARM templates, which means anything that can be don in an ARM Template can be done in bicep.
- Bicep files are compiled to standard ARM Template JSON files
- Bicep CLI is written with .net core (Mac, Linux and Windows versions available)
- The current version is 0.1
- Really early preview
Now is a perfect time to go and comment or contribute into the features!
Tooling
To get the best bicep authoring experience, you will need two components:
- Bicep CLI (required) - Compiles bicep files into ARM templates. Cross-platform.
- Bicep VS Code Extension - Authoring support, intellisense, validation. Optional, but recommended.
You need the Bicep CLI which does the compilation, and the VS code plugin comes in handy which provides you intellisence!
The current version is number 0.1 -> it is published very RAW, so we can all contribute from the beginning!
Installing Bicep CLI (Powershell)
# Create the install folder
$installPath = "$env:USERPROFILE\.bicep"
$installDir = New-Item -ItemType Directory -Path $installPath -Force
$installDir.Attributes += 'Hidden'
# Fetch the latest Bicep CLI binary
(New-Object Net.WebClient).DownloadFile("https://github.com/Azure/bicep/releases/latest/download/bicep-win-x64.exe", "$installPath\bicep.exe")
# Add bicep to your PATH
$currentPath = (Get-Item -path "HKCU:\Environment" ).GetValue('Path', '', 'DoNotExpandEnvironmentNames')
if (-not $currentPath.Contains("%USERPROFILE%\.bicep")) { setx PATH ($currentPath + ";%USERPROFILE%\.bicep") }
if (-not $env:path.Contains($installPath)) { $env:path += ";$installPath" }
# Verify you can now access the 'bicep' command.
bicep --help
# Done!
Installing the Bicep VS Code extension (Windows PowerShell)
# Fetch the latest Bicep VSCode extension
$vsixPath = "$env:TEMP\vscode-bicep.vsix"
(New-Object Net.WebClient).DownloadFile("https://github.com/Azure/bicep/releases/latest/download/vscode-bicep.vsix", $vsixPath)
# Install the extension
code --install-extension $vsixPath
# Clean up the file
Remove-Item $vsixPath
# Done!
How bicep looks like
First, verify the Bicep CLI and VS Code extension are running.
Let’s start by creating a blank file main.bicep. If you create the main.bicep file in VS code and extension is installed, you should see syntax highlighting working, and you should see the language mode in the lower right-hand corner of the VS code window change to bicep.
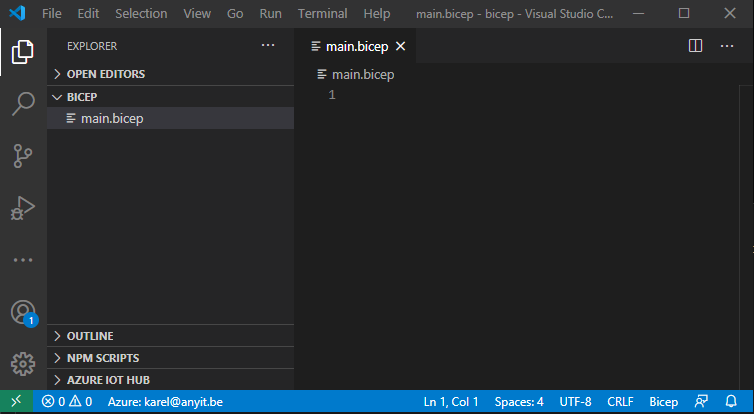
Next, compile the bicep file by running:
bicep build main.bicep
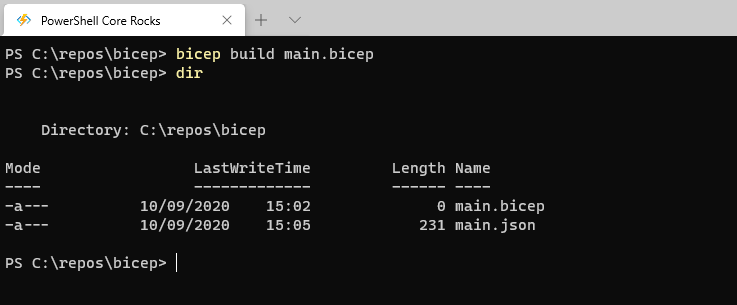
After compiling an empty Bicep file, it will automatically include the basic JSON syntax of the ARM Template. When you compile an empty Azure Bicep file, the resulting ARM Template will include only this basic JSON outline.
{
"$schema": "https://schema.management.azure.com/schemas/2019-04-01/deploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {},
"functions": [],
"variables": {},
"resources": [],
"outputs": {}
}
Bicep sample
Let me explain the simple example you can find below:
- First it says that this is a resource
- stg is a symbolic name. This is an identifier for referencing the resource throughout your bicep file.
- type is like we know it in ARM, composed of the resource provider (Microsoft.Storage), resource type (storageAccounts), and apiVersion (2019-06-01)
- Then we have the properties (everything inside = {…}). These are properties like the name of the resource, the location, kind and sku
- You can put comments in single line or multi line comments if you want
resource stg 'Microsoft.Storage/storageAccounts@2019-06-01' = {
name: 'kdwtbicepstorage01' // must be globally unique
location: 'westeurope'
kind: 'Storage'
sku: {
name: 'Standard_LRS'
}
}
You can also add parameters, variables and outputs. For example, we could also define output, like storage ID or storage name.
Bicep sample output
When you compile the Azure Bicep file, you get the ARM template as the output.
{
"$schema": "https://schema.management.azure.com/schemas/2019-04-01/deploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {},
"functions": [],
"variables": {},
"resources": [
{
"type": "Microsoft.Storage/storageAccounts",
"apiVersion": "2019-06-01",
"name": "kdwtbicepstorage01",
"location": "westeurope",
"kind": "Storage",
"sku": {
"name": "Standard_LRS"
}
}
],
"outputs": {}
}
Deploying a bicep file
Bicep files cannot yet be directly deployed via the Az CLI or PowerShell Az module. You must first compile the bicep file with bicep build then deploy via deployment commands (az deployment group create or New-AzResourceGroupDeployment).
What about complex bicep files
If you have done the complex concatenation of an ARM template, you know that it is hard.
The concatenation of the strings in a bicep file is more simpler!
The bicep file is more simpler and easy to use! It is going to be closer to Powershell then a ARM template is. Because people know that editing ARM templates is hard.
Conclusions
Is Azure Bicep the future? I think for most of the people it depends. If you have already made complex ARM Templates and invested a lot of time, I don’t think you would go and learn a new language. Just because you already have the skills and master the creation of ARM Templates.
On the other hand, if you are new to Azure, it will be easier to use and maintain bicep because of simplicity.
Maybe when we look back within a year (or maybe 2) from now, it will be ready to use, because it is not ready today. Within a year Azure bicep will be good and I hope in 2 years, this will be great!
ARM is not death
You also have to know that Microsoft is putting big amounts of effort into ARM. There’s more effort from Microsoft side on improving ARM than we previously knew. How? Well, we can see that because ARM is getting cool new features and is still improving.
Introducing something new like Bicep is a huge effort of Microsoft. They need to rework all ARM exports from the portal, write documentation, change references and so on.
There is a huge amount of people working on it! So keep an eye on it, because it will be big!
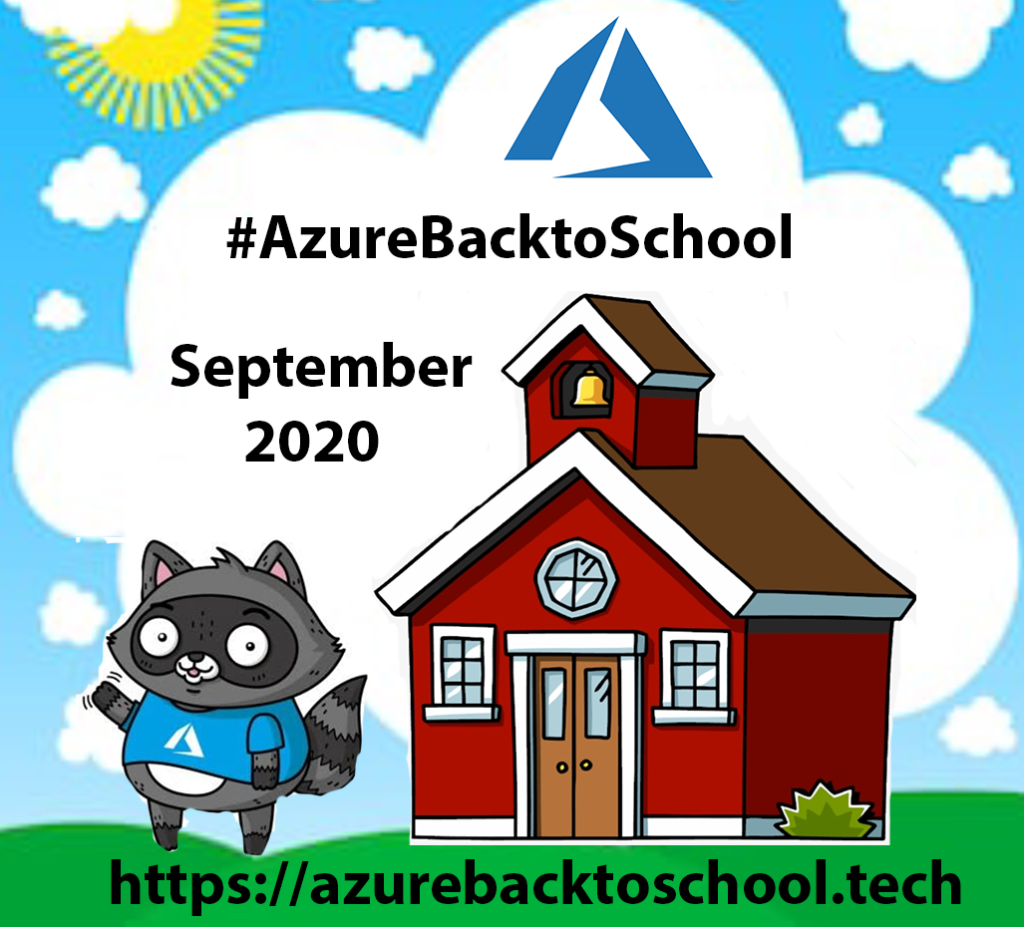
Don’t forget the check the upcomming articles from #AzureBackToSchool!